Linux Kernel and Drivers Development
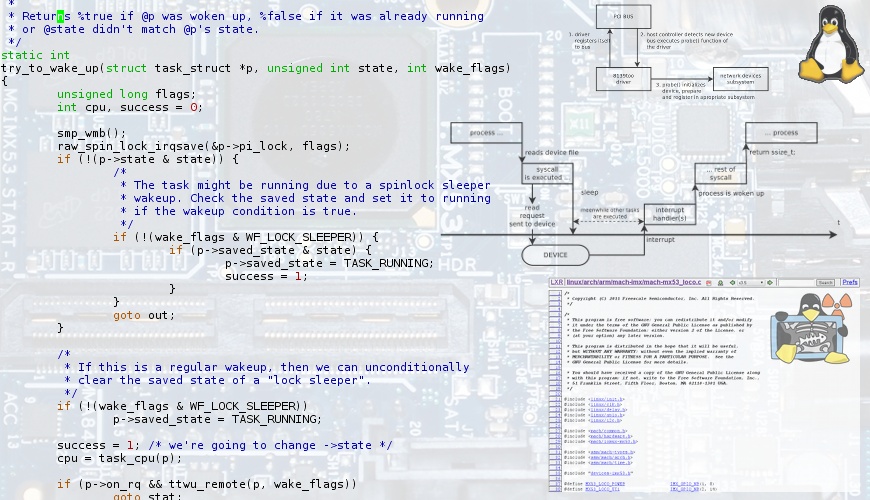
This intensive course introduces you to kernel programming techniques and framework. An ARM machine is used as a hardware platform, on which variety of kernel interfaces, frameworks, and device drivers are explored and developed. The course is intended for engineers who often need to modify, create, or just test the Linux kernel code in their everyday work.
Course outline
- Introduction to kernel programming, preparing test system and configuring environment.
- Kernel modules
- memory management and IO
- processes and scheduler
- VFS, file operations
- time
- interrupts
- interrupt-based input-output
- multithread synchronization
- Linux device model with examples
- Network devices
- Block devices
- Input subsystem
- PCI
- DMA
- Kernel debugging techniques
During the practical exercises, the participants will develop test and debug a device driver for a real hardware connected to I2C and GPIO lines.
Detailed agenda
Day 1
- Introduction to kernel programming, preparing test system and configuring environment.
- Kernel modules -- various kernel code examples and exercises. Integrating and maintaining code.
- memory management: address space, virtual memory, memory allocation:
kmalloc
, kzalloc
, vmalloc
, get_free_pages
, ioremap
, page allocators (SLAB, SLUB, SLOB), low memory conditions -- oom_killer
.
- IO methods, implementing driver (serial device driver) from silicon specification.
- processes: virtual memory, process address spaces kernel address space, process lifecycle, process creation (from userspace and from kernel), threads (NPTL and linuxthreads)
- scheduler: O(1) and CFQ process schedulers, cgroup scheduler, scheduling classes:
SCHED_OTHER
, SHCED_FIFO
, SCHED_RR
and other, task_struct
, process handling and parameters, cpu affinity.
- VFS, advanced file operations: userspace to kernelspace communication (synchronic and asynchronous functions), character devices,
ioctl
, mmap
, AIO.
- Time in kernel: measuring time lapse and cycles, sleeping:
usleep
, nanosleep
, other methods timers, timer drivers for embedded devices, methods of sleeping and suspending code execution, high resolution timers.
- Interrupts: interrupt handler registration, passing data from interrupt context to userspace, deferred interrupt handling (bottom halves): softirqs, tasklets, workqueues, interrupts as threads (real-time aspects of irq latency). ARM: IRQ vs. FIQ.
During the 'Day 1' participants gain the broad knowledge about operations of Linux kernel and its internals. They will get familiar with kernel programming and configurations. They run and test on their own variety of drivers in an emulated embedded environment. Exercises will cover most of kernel -- userspace communication and basic in-kernel programming frameworks, creating base for next work.
Day 2
- interrupts-based input-output: methods of process-interrupt synchronization, waitqueues, real driver example
- multitasking and synchronization: problems and solutions, atomic variables, mutexes, semaphores, spinlocks, conditionals, non-blocking mechanisms -- RCU.
- Linux unified device model: how system sees the hardware, system boot and SoC bring-up, bus, bus driver, bus controller, driver, device, automatic driver matching, various busses: USB, PCI, Platform, registering device driver, passing data, control interfaces in /sys and /proc. Platform devices and Device-Tree
- Network devices: drivers structure, network transmission control, socket interface, drivers optimizations.
- Block devices: drivers structure and performance.
- Input subsystem: drivers structure, device handling
- PCI: driver structure, driver registration, device handling, special PCI functions.
- Framebuffer -- device structure, options and operations, SPI framebuffer.
After 'Day 2', participants will be familiar with (most important in day-to-day work) Linux unified device model framework and interrupt controlled input-output devices. This will give them knowledge about operations of most important kernel subsystems. Real code examples will be generated and tested, various device drivers will be examined.
Day 3
- DMA: memory allocation for DMA transactions, synchronization, DMA-API.
- RT and latency aspects in Linux kernel: latency in standard kernel, kernel preemption, additional real-time solutions: RT_PREEMPT, Xenomai
- Kernel debugging techniques: tools and techniques, external and internal debugging methods, JTAG, QEMU JTAG-like emulation, kernel tracers (ftrace), kernel profiling.
- Memory leaks debugging, tools, tips'n'tricks, KGDB, debugging by querying, real-life examples.
Most important part of the 'Day 3' is kernel debugging hands-on exercise. Participants will not only know how the kernel works, but also will learn to cope with problems and bugs in own and external code. Some aspects of real-time operations, kernel optimizations, and profiling will be also mentioned and practiced.