Embedded Linux Development
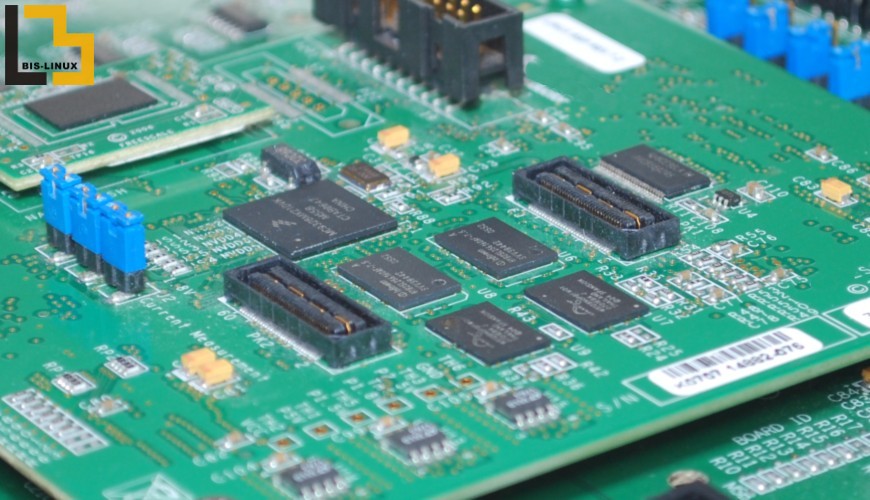
Developers building embedded solutions using Linux often need to make certain decisions and complete tasks such as: selecting the proper toolchain, software versions, changing and adding some kernel code (device drivers) and system code, and also evaluating the time needed to deliver working solution. This advanced course will provide you with the knowledge and experience needed to use Linux within your project (regardless whether you are already using it, or just evaluating).
You will gain a complete understanding of all aspects of building and deploying an embedded Linux system: from boot-loader and system boot-strapping, through kernel drivers programming, and code organization, system level programming, to creating GUI and network embedded applications, making use of high-level open source software and finally debugging, profiling and optimizing system. Lots of code examples are provided too.
Through hands-on exercises, you will gain experience of device drivers, protocols and components often found in embedded systems.
Course outline
- Linux embedded systems fundamentals
- Setting an embedded board, board bring-up, building, configuring, and running a bootloader, Linux kernel and base system on it.
- Cross-Development environments: goals, needs, techniques, Buildroot and Yocto Project based automatic build environments.
- Root filesystem choices and best practices. Flash memory and embedded filesystems.
- Linux device model and kernel debugging. Device-Tree.
-
- Specific kernel subsystems for embedded: Serial, I2C, SPI, Framebuffer (display), USB, GPIO.
- Userspace configuration, process and resource management: systemd, units, services, hotplug and device configuration.
- System programming and userspace applications with examples: multimedia, scripts etc..
- Using development tools (Eclipse, VSCode) to develop and debug Embedded Linux applications.
During this intensive training more subjects are discussed with corresponding examples, such as: licenses, ownage and control of open-source code, system security and even some aspects of Linux administration (needed in embedded context) as well as maintaining best practices.
Detailed agenda
1. Linux embedded systems fundamentals
- introduction to Embedded Linux system and its components, hardware requirements and design principles,
- cross-compiler - design and planning, common design decisions and pitfalls (binary toolchain vs. self-build one)
- building, configuring and running simple Linux system for embedded from scratch: bootloader
u-boot
,
- Linux kernel and its configuration - configuring kernel, kernel programming preview, working with kernel source code
- configuring base system (Busybox), its configuration and scripts
- boot process profiling, boot time optimization.
After 'Part 1'
Participants will be able to select proper tool-chain for a task, prepare simple Linux system: configure kernel, select drivers and mechanisms, configure it: create boot scripts, configure standard system services (such as network connectivity) and security (users and permissions). Participants will gain a hands-on familiarity with essential components of Embedded Linux stack and understand their relationships.
Exercises
During this day's series of practical exercises the participants configure, compile from scratch and experiment with building blocks of Linux Embedded system.
- Cross-compiler - how to use it (paths, sysroot, tools, additional libraries).
- Bootloader source code configruation, compilation and running. Modifying
u-boot
sourcecode. Using bootloader to bring-up the device.
- Cross-compilation of Linux kernel source code. Booting the kernel - typical errors and caveats.
- Using solid-state memory to store firmware - booting from SD cart/eMMC/NAND flash.
- Root filesystem (Busybox), startup scripts, components, configuration, basic system configuration scripts.
- Debugging typical board bring-up problems and boot process.
- (optional): Fine tuning the cross-toolchain. Using
crosstool-ng
to build toolchains from scratch. Typical problems and solutions.
2. Building and debugging system as a whole using Yocto Project. Root filesystem strategies. Introduction to kernel development
- Buildroot - a buildsystem at a first glance - automating tasks from day 1.
- Yocto Project - a sophisticated build ecosystem,
- Root filesystem strategies (ramdisk vs. solid-state)
- block devices and filesystems: ext4, XFS (with real-time), squashfs
- flash memories and filesystems: JFFS2, YAFFS, UBI, flash transaction layer.
- automatic and manual system update approach (
SWUpdate
, hawkbit
, Mender.io
)
- Embedded Linux development using IDE (Eclipse, VSCode)
- introduction to system programming
- introduction to device drivers programming
- in - kernel programming (characteristic, legal issues -- licenses, view from userspace)
- kernel modules (common container for code)
- kernel-space - userspace interaction - methods of accessing drivers data from userspace (from configuration flags to big data chunks): procfs, sysfs, debugfs, device files, mmap.
- proces management, memory, timers, synchronization and interrupts
- Linux device model - devices: character, block and network devices (all Unix device types); buses (bus drivers), drivers and devices management and coupling.
After 'Part 2'
All about Linux kernel programming is the layer based design and framework. Participants will understand the design of Linux kernel and will be able to extend code (adding and adjusting drivers) as well as to select a proper layer and framework for a given task (not all tasks need in-kernel programming, some can be resolved totally in userspace). Many code snippets and working examples will be presented.
Exercises
- (optional) Developing the Embedded Linux system using Buildroot.
- Developing the Embedded Linux system using Yocto Project (distribution configuration, tools and how to use them, design principles, building images, adding additional meta-layers and packages).
- (optional) using network filesystem (NFS) for development.
- Filesystems for block devices and NAND Flash devices.
- Software update strategies (
SWUpdate
based update system).
- Using Eclipse or VSCode to cross-compile applications and linux kernel modules. Deploying, running and debugging the code - cross compilation and working with remote system on a board. Best practices of remote development and debugging.
- Compiling and using kernel modules (as a driver container). Out-of tree driver development introduction.
Part 3. Linux kernel device model. Userspace programs - Systemd. Embedded Linux debugging and profiling
- How does Linux see hardware? - Input-output polling and interrupts.
- Linux device model with working examples. Frameworks and internal kernel API.
- Device-Tree - platform driver description format.
- Real-life appliances of device frameworks: USB, I2C, 1-wire, SPI, network devices, DMA, PWM.
- Selected topics of kernel debugging.
systemd
- userspace processes management
udev
- hardware detestion and configuration
- start-up rpoces and tasks management (
unit
, service
)
- network interface and network address configuration
- resources management (CPU cycles, memory, system bus throughput,
namespaces
)
- Linux Kernel and userspace processes profiling and tracing
- System tracing using graphical tools, boot time profiling
After 'Part 3'
Today nobody implements drivers by creating code for all its layers (from userspace interaction to hardware handling) as there are frameworks for almost every driver.
The complete system will be deployed to a development board. Participants will be given overview of potentially interesting tools and technologies. Again, with numerous working examples and code snippets, such as: embedded controller with GUI in QT, embedded measuring device with network access and control via WEB interface, wireless connected device, multimedia appliances, and so on.
Exercises
This series of exercises contains lots of examples of scripts, source code and applications to be modified and run on the device.
All the boilerplate is ready, the participant needs to modify the code, add important function or modify the code to perform an experiment.
- Device-Tree - configuring and describing the hardware for a driver (example of 1-wire or LED device).
- working with board schematics, SoC configuration: multiplexing and PAD control. Typical problems and solutions.
- Using
systmd
to configure base system and application. System configuration: udev
, units
, services
, devices
.
- Userspace - kernel configuration
- remote application debugging - example aplication RTSP server.
- configuring
systemd
to automatically bring-up the application and restart it on failure, watchdog.
- automatic configuration of network devices, WiFi, USB endpoint etc.